Realm Database
Used by 100k+ developers and downloaded over 2 billion times. Realm Database is a fast, easy to use, and open source alternative to SQLite and Core Data.
Start Building Now
Pick you flavor of the Realm's offline-first database below and reach out on our forums if you have any questions.
class Dog {}
Dog.schema = {
name: 'Dog',
properties: {
name: 'string',
age: 'int',
}
};
let realm = new Realm({ schema: [Dog] });
realm.write(() => {
realm.create('Dog', {name: 'Rex', age: 1});
});
let pups = realm.objects('Dog').filtered('age < 2');
Dog *dog = [Dog new];
dog.name = @"Rex";
dog.age = 1;
RLMRealm *realm = [RLMRealm defaultRealm];
[realm transactionWithBlock:^{
[realm addObject:dog];
}];
RLMResults<Dog *> *allDogs = [Dog allObjects];
RLMResults<Dog *> *pups = [allDogs objectsWhere:@"age < 2"];
class Dog: Object {
@objc dynamic var name = ""
@objc dynamic var age = 0
}
let dog = Dog()
dog.name = "Rex"
dog.age = 1
let realm = try! Realm()
try! realm.write {
realm.add(dog)
}
let pups = realm.objects(Dog.self).filter("age < 2")
public class Dog extends RealmObject {
public String name;
public int age;
}
Dog dog = new Dog();
dog.name = "Rex";
dog.age = 1;
Realm realm = Realm.getDefaultInstance();
realm.beginTransaction();
realm.copyToRealm(dog);
realm.commitTransaction();
RealmResults<Dog> pups = realm.where(Dog.class)
.lessThan("age", 2)
.findAll();
public class Dog : RealmObject
{
public string Name { get; set; }
public int Age { get; set; }
}
var realm = Realm.GetInstance();
realm.Write(() =>
{
realm.Add(new Dog
{
Name = "Rex",
Age = 1
});
});
var pups = realm.All<Dog>().Where(d => d.Age < 2);
Simplify your code Just subclass RealmObject to define your schema, subclass RLMObject to define your schema, define your schema, subclass Object to define your schema, subclass RealmObject to define your schema, persist with lightweight transactions, and query using fluent interfaces. NSPredicate. simple strings. NSPredicate. LINQ. Working with your data should always be this easy.
Just subclass RealmObject to define your schema, subclass RLMObject to define your schema, define your schema, subclass Object to define your schema, subclass RealmObject to define your schema, persist with lightweight transactions, and query using fluent interfaces. NSPredicate. simple strings. NSPredicate. LINQ. Working with your data should always be this easy.
React Native executes JavaScript in a dedicated worker thread so common concurrency primitives are unavailable.
// Query and update the result in another thread
dispatch_async(dispatch_queue_create("background", 0), ^{
Dog *theDog = [[Dog objectsWhere:@"age == 1"] firstObject];
RLMRealm *realm = [RLMRealm defaultRealm];
[realm beginWriteTransaction];
theDog.age = 3;
[realm commitWriteTransaction];
});
// Query and update from any thread
DispatchQueue(label: "background").async {
let realm = try! Realm()
let theDog = realm.objects(Dog.self).filter("age == 1").first
try! realm.write {
theDog!.age = 3
}
}
public void onActivityCreated(Bundle savedInstanceState) {
realm = Realm.getDefaultInstance();
customers = realm.where(Customer.class).findAllAsync();
changeListener = new RealmChangeListener() {
@Override
public void onChange(RealmResults<Customer> results) {
listAdapter.notifyDataSetChanged(); // Update the UI
}
};
customers.addChangeListener(changeListener);
}
// Query and update from any thread
Task.Run(() =>
{
var realm = Realm.GetInstance();
var theDog = realm.All<Dog>().First(d => d.Age == 1);
realm.Write(() => theDog.Age = 3);
});
Work across threads To start work on a new thread, just get a new reference to your Realm, and everything else just works. Each thread always has a consistent view of the data, which means illegal states are impossible.
To start work on a new thread, just get a new reference to your Realm, and everything else just works. Each thread always has a consistent view of the data, which means illegal states are impossible.
let key = new Int8Array(64); // pupulate with a secure key
let realm = new Realm({ schema: [Dog], encryptionKey: key });
let dogs = realm.objects('Dog').filtered('name CONTAINS "Fido"');
// Generate a random encryption key
NSMutableData *key = [NSMutableData dataWithLength:64];
(void)SecRandomCopyBytes(kSecRandomDefault, key.length, (uint8_t *)key.mutableBytes);
// Open the encrypted Realm file
RLMRealmConfiguration *config = [RLMRealmConfiguration defaultConfiguration];
config.encryptionKey = key;
RLMRealm *realm = [RLMRealm realmWithConfiguration:config error:nil];
// Use the Realm as normal
RLMResults<Dog *> *dogs = [Dog objectsInRealm:realm where:@"name contains 'Fido'"];
// Generate a random encryption key
var key = Data(count: 64)
_ = key.withUnsafeMutableBytes { bytes in
SecRandomCopyBytes(kSecRandomDefault, 64, bytes)
}
// Open the encrypted Realm file
let realm = try! Realm(configuration: Realm.Configuration(encryptionKey: key))
// Use the Realm as normal
let dogs = realm.objects(Dog.self).filter("name contains 'Fido'")
// Generate a random encryption key
byte[] key = new byte[64];
new SecureRandom().nextBytes(key);
// Open the encrypted Realm file
RealmConfiguration realmConfiguration = new RealmConfiguration.Builder()
.encryptionKey(key)
.build();
// Use the Realm as normal
Realm realm = Realm.getInstance(config);
RealmResults<Dog> results = realm.where(Dog.class).contains("name", "Fido").findAll();
// Generate a random encryption key
var random = new Random();
var key = new Byte[64];
rnd.NextBytes(key);
var config = new RealmConfiguration("Mine.realm");
config.EncryptionKey = key;
// Open the encrypted Realm file
var realm = Realm.GetInstance(config);
// Use the Realm as normal
var dogs = realm.All<Dog>().Where(d => d.Name.Contains("Fido"));
Encrypt everything Keep your users’ data safe with AES-256 bit encryption, strong enough for global financial institutions.
Keep your users’ data safe with AES-256 bit encryption, strong enough for global financial institutions.
let realm = new Realm({ schema: [Person] });
realm.addListener((sender, name) => {
if (name === 'change') {
this.setState({ source: sender.objects('Dog') });
}
});
self.notificationToken = [[Dog objectsWhere:@"age > 5"]
addNotificationBlock:^(RLMResults<Dog *> *results,
RLMCollectionChange *change,
NSError *error) {
if (error) {
// handle error
return;
} else if (!changes) {
[self.tableView reloadData];
return;
}
// Update table view to animate deletions, insertions and modifications
[tableView beginUpdates];
[tableView deleteRowsAtIndexPaths:[changes deletionsInSection:0]
withRowAnimation:UITableViewRowAnimationAutomatic];
[tableView insertRowsAtIndexPaths:[changes insertionsInSection:0]
withRowAnimation:UITableViewRowAnimationAutomatic];
[tableView reloadRowsAtIndexPaths:[changes modificationsInSection:0]
withRowAnimation:UITableViewRowAnimationAutomatic];
[tableView endUpdates];
}];
let realm = try! Realm()
notificationToken = realm.objects(Dog.self).filter("age > 5").observe { changes in
switch changes {
case .initial:
tableView.reloadData()
break
case .update(_, let deletions, let insertions, let modifications):
// Update table view to animate deletions, insertions and modifications
break
case .error(let error):
// handle error
break
}
}
Realm realm = Realm.getDefaultInstance();
// Query in the background
RealmResults<Dog> results = realm.where(Dog.class)
.greaterThan("age", 5)
.findAllAsync();
// Use ChangeListeners to be notified about updates
results.addChangeListener(new RealmChangeListener<RealmResults<Dog>() {
@Override
public void onChange(RealmResults<Dog> results) {
// Update UI
}
});
// Or RxJava
Observable<RealmResults<Dog>> obs = results.asObservable();
// To bind a ListView to results of a query
public partial class DogsPage : ContentPage
{
internal Realm realm;
internal IEnumerable<Dog> Entries { get; }
public DogsPage()
{
InitializeComponent();
realm = Realm.GetInstance();
// Results from queries will automatically notify the Binding
// and update the ListView accordingly
Entries = realm.All<Dog>().Where(d => d.Age > 5)
DogsList.ItemsSource = Entries;
}
<ListView x:Name="DogsList">
<ListView.ItemTemplate>
<DataTemplate>
<TextCell Text="{Binding Name}"
Detail="{Binding Age, StringFormat='is {0:#}'}" />
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
Build reactive apps with live objects Realm objects are always live, which means that they always have the latest data. Subscribe to notifications to get updates when data changes, then update your UI. Your app will never be out of date again.
Realm objects are always live, which means that they always have the latest data. Subscribe to notifications to get updates when data changes, then update your UI. Your app will never be out of date again.
// Authenticating the User
Realm.Sync.User.registerWithProvider('http://realm.example.co:9080', 'google', googleAccessToken, (error, user) => {
if (!error) {
// Opening a remote Realm
var realm = new Realm({
sync: {
user: user,
url: 'realm://realm.example.co:9080/~/userRealm',
}
});
// Any changes made to this Realm will be synced across all devices!
}
})
// Authenticating the User
[RLMSyncUser logInWithCredentials:[RLMSyncCredentials credentialsWithGoogleToken:@"google token"]
authServerURL:[NSURL URLWithString:@"http://realm.example.com:9080"]
onCompletion:^(RLMSyncUser *user, NSError *error) {
if (user) {
// Opening a remote Realm
NSURL *realmURL = [NSURL URLWithString:@"realm://realm.example.com:9080/~/userRealm"];
RLMRealmConfiguration *config = [[RLMRealmConfiguration alloc] init];
config.syncConfiguration = [[RLMSyncConfiguration alloc] initWithUser:user realmURL:realmURL];
RLMRealm *realm = [RLMRealm realmWithConfiguration:config error:nil];
// Any changes made to this Realm will be synced across all devices!
} else if (error) {
// handle error
}
}];
// Authenticating the User
SyncUser.logIn(with: .google(token: "google token"),
server: URL(string: "http://realm.example.com:9080")!) { user, error in
if let user = user {
// Opening a remote Realm
let realmURL = URL(string: "realm://realm.example.com:9080/~/userRealm")!
let realm = try! Realm(configuration: user.configuration())
// Any changes made to this Realm will be synced across all devices!
} else if let error = error {
// handle error
}
}
// Authenticating the User
User user = User.login(Credentials.google("google token"),
"http://realm.example.com:9080/auth");
// Opening a remote Realm
String realmURL = "realm://realm.example.com:9080/~/userRealm";
Realm realm = Realm.getInstance(user.configuration());
// Any changes made to this Realm will be synced across all devices!
var user = await User.LoginAsync(Credentials.Google("google token"),
new Uri("http://realm.example.com:9080"));
var realmUrl = new Uri("realm://realm.example.com:9080/~/userRealm");
var realm = Realm.GetInstance(user.configuration());
// Any changes made to this Realm will be synced across all devices!
Sync data seamlessly With the Realm Platform extensions, the Realm Database works perfectly with the Platform. All you need to do is set your Realm Platform Instance URL; everything else stays exactly the same. Data sync will automatically happen whenever you save, with no work from you.
Available for Java, Swift, Objective‑C, JavaScript, and Xamarin.
With the Realm Platform extensions, the Realm Database works perfectly with the Platform. All you need to do is set your Realm Platform Instance URL; everything else stays exactly the same. Data sync will automatically happen whenever you save, with no work from you.
Available for Java, Swift, Objective‑C, JavaScript, and Xamarin.
Benefits of a database built for mobile
Realm Studio
Realm Studio is our premiere developer tool, built so you can easily manage the Realm Database and Realm Platform. With Realm Studio, you can open and edit local and synced Realms, and administer any Realm Object Server instance. Download it now on macOS, Windows, or Linux.
Read a Whitepaper
Learn about Realm's best practices, approach to identity, access control, auditing and logging and encryption. Check out some of our whitepapers below.
Read moreTry a Demo
Learn how to get a synchronized Realm up and running with just 20 lines of code, and discover the power of server-side event handling in a simple demo.
Try a DemoWatch a Webinar
Get a clear and practical overview of the Realm Platform and its top use cases in these presentations and demos.
Watch nowGet Started with Realm
Trusted by Fortune 500 mainstays, innovative startups, and #1-ranked app store successes
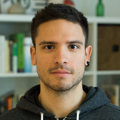
Me using @realm for the first time: 'this CAN’T be that easy. Pretty sure I’m missing something.'
Marco Sero
iOS Engineer @ Google
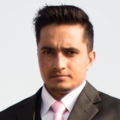
I boosted my database of 200,000 rows search from 7 seconds to 0.5 seconds with @realm . Practically under 30 mins of using it 1st time.
Vijaya P. Kandel
iOS Engineer
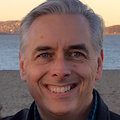
Very impressive! I’m a huge fan of innovative solutions—especially critical processes like data persistence. 💯
Kevin Hoctor
Software Engineer @ Apple